In this example we will create a temperature and humidity meter using a sht31 sensor and display the readings on an LCD shield
SHT31 is the next generation of Sensirion’s temperature and humidity sensors. It builds on a new CMOSens® sensor chip that is at the heart of Sensirion’s new humidity and temperatureplatform.
The SHT3x-DIS has increased intelligence, reliability and improved accuracy specifications compared to its predecessor. Its functionality includes enhanced signal processing, two distinctive and user selectable I2C addresses and communication speeds of up to 1 MHz. The DFN package has a footprint of 2.5 x 2.5 mm2 while keeping a height of 0.9 mm.
This allows for integration of the SHT3x-DIS into a great variety of applications.
Features
Fully calibrated, linearized, and temperature compensated digital output
Wide supply voltage range, from 2.4 V to 5.5 V
I2C Interface with communication speeds up to 1 MHz and two user selectable addresses
If you’re using an Arduino simply connect the VIN pin to the 5V or 3.3v voltage pin, GND to ground, SCL to I2C Clock (Analog 5) and SDA to I2C Data (Analog 4).
Parts List
Description | Link |
Arduino Uno | 1pcs UNO R3 CH340G+MEGA328P for Arduino UNO R3 (NO USB CABLE) |
LCD Keypad shield | 1PCS LCD Keypad Shield LCD 1602 Module Display For Arduino |
SHT31 sensor | SHT31 Temperature Humidity Sensor module Breakout Weather for Arduino |
connecting wire | 40pcs Dupont jumper wire cable 30cm male to male,female to female,male to female Dupont jump wire line 2.54mm breadboard cable |
Connection
In this example we show the connections to a typical LCD shield
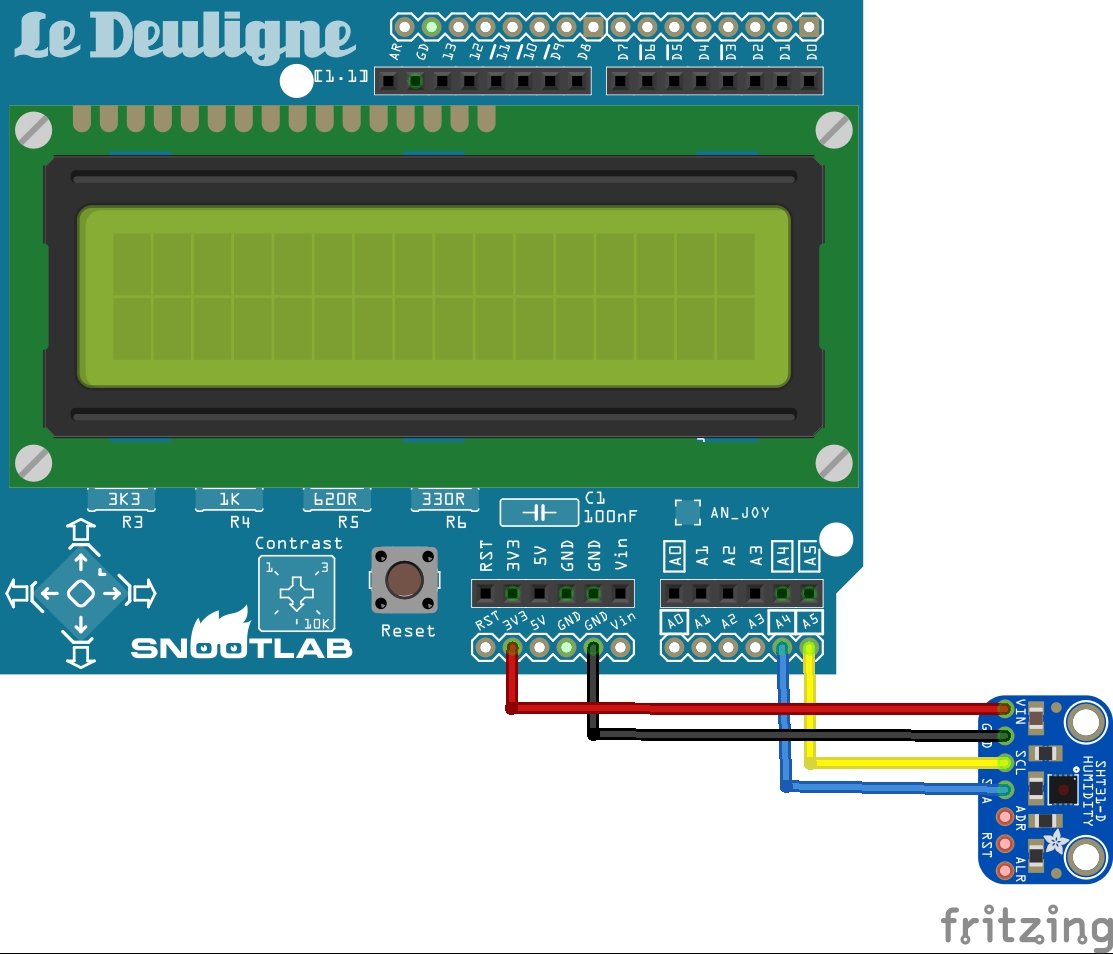
lcd shield and sht31 layout
Code
You need to add the adafruit sht31 library, this is available in the library manager
[codesyntax lang=”cpp”]
#include <Wire.h> #include <LiquidCrystal.h> #include "Adafruit_SHT31.h" //setup for the LCD keypad shield LiquidCrystal lcd(8, 9, 4, 5, 6, 7); Adafruit_SHT31 sht31 = Adafruit_SHT31(); void setup() { //init serial for some debug Serial.begin(9600); Serial.println("Light Sensor Test"); //init sht31 if (! sht31.begin(0x44)) { Serial.println("Couldn't find SHT31"); while (1) delay(1); } //init LCD lcd.begin(16,2); //line 1 - temperature lcd.setCursor(0,0); lcd.print("Temp = "); //line 2 - humidity lcd.setCursor(0,1); lcd.print("Hum = "); } void loop() { // put your main code here, to run repeatedly: float t = sht31.readTemperature(); float h = sht31.readHumidity(); //display temperature on lcd if (! isnan(t)) { lcd.setCursor(0,0); lcd.print("Temp = "); lcd.print(t); } else { Serial.println("Failed to read temperature"); } //display humidity on lcd if (! isnan(h)) { lcd.setCursor(0,1); lcd.print("Hum = "); lcd.print(h); } else { Serial.println("Failed to read humidity"); } Serial.println(); delay(500); }
[/codesyntax]
Link