In this article we create a distance measurement ultrasonic project, we will use an Arduino Uno with an LCD Keypad shield. We will then connect the HY-SFR05 to this shield and display the readings on the LCD. Lets go and look at the ultrasonic sensor
The HY-SRF05 is an ultrasonic emitter/receiver used to measure distance with a precision of ~0.3cm. It sends out a 40 KHz square wave signal that reflects on objects in front of the sensor. This signal is then read back by the sensor and the duration of the received signal is reflected on the ECHO pin.
Features
Supply voltage: 4.5V to 5.5V
Supply current: 10 to 40mA
Trigger pin format: 10 uS digital pulse
Sound frequency: 40 KHz
Echo pin output: 0V-VCC
Echo pin format: digital
How to use
Send a 10Us wide pulse (low to high) to the Trigger pin.
Monitor the ECHO pin.
When the ECHO pin goes HIGH, start a timer.
When the ECHO pin goes LOW, stop the timer and save the elapsed time.
Use the elapsed time in the following formula to get the distance in cm:
Distance (in cm) = (elapsed time * sound velocity (340 m/s)) / 100 / 2
We will see a code example later
Parts List
Name | Link |
Arduino Uno | UNO R3 CH340G/ATmega328P, compatible for Arduino UNO |
HY-SRF05 | HY-SRF05 SRF05 Ultrasonic Ranging Module Ultrasonic Sensor |
LCD Keypad shield | 1PCS LCD Keypad Shield LCD 1602 Module Display For Arduino |
connecting wire | Free shipping Dupont line 120pcs 20cm male to male + male to female and female to female jumper wire |
Layout
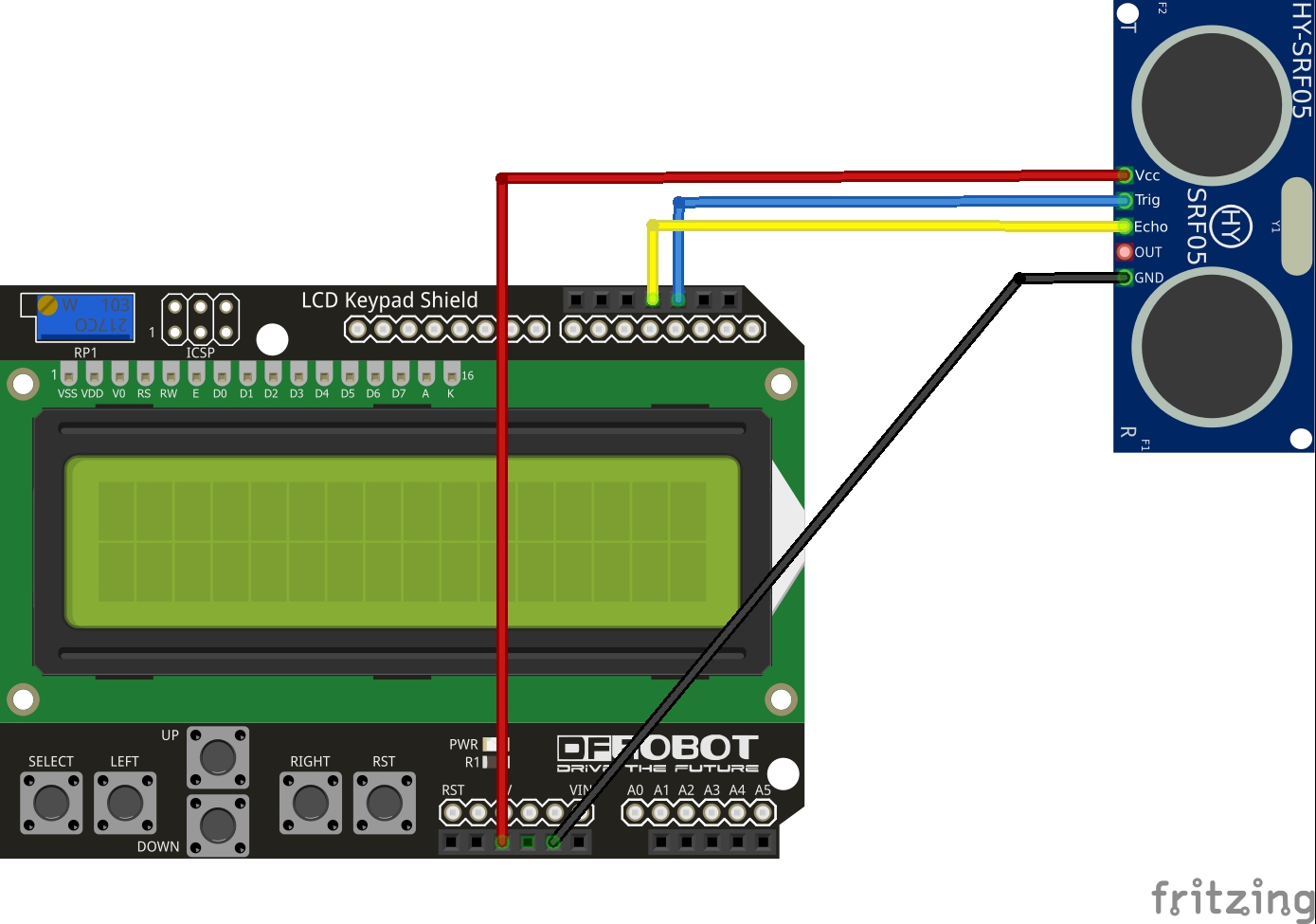
arduino and lcd keypad shield and HY-SRF05
Code
[codesyntax lang=”cpp”]
#include <Wire.h> #include <LiquidCrystal.h> //setup for the LCD keypad shield LiquidCrystal lcd(8, 9, 4, 5, 6, 7); const int TRIG_PIN = 2; const int ECHO_PIN = 3; void setup() { pinMode(TRIG_PIN,OUTPUT); pinMode(ECHO_PIN,INPUT); //init LCD lcd.begin(16,2); //line 1 - temperature lcd.setCursor(0,0); lcd.print("Distance"); } void loop() { long duration, distanceCm, distanceIn; digitalWrite(TRIG_PIN, LOW); delayMicroseconds(2); digitalWrite(TRIG_PIN, HIGH); delayMicroseconds(10); digitalWrite(TRIG_PIN, LOW); duration = pulseIn(ECHO_PIN,HIGH); // convert the time into a distance distanceCm = duration / 29.1 / 2 ; distanceIn = duration / 74 / 2; lcd.setCursor(0,0); if (distanceCm <= 0) { lcd.print(distanceCm); lcd.print("???"); } else { //display readings on lcd lcd.clear(); lcd.print("Distance: "); lcd.print(distanceCm); lcd.print("cm"); } lcd.print(""); delay(500); }
[/codesyntax]