Another temperature project, this time we will use a TMP102 to an LCD keypad shield
The TMP102 device is a digital temperature sensor ideal for NTC/PTC thermistor replacement where high accuracy is required. The device offers an accuracy of ±0.5°C without requiring calibration or external component signal conditioning. Device temperature sensors are highly linear and do not require complex calculations or lookup tables to derive the temperature. The on-chip 12-bit ADC offers resolutions down to 0.0625°C.
The TMP102 device features SMBus™, two-wire and I2C interface compatibility, and allows up to four devices on one bus. The device also features an SMBus alert function. The device is specified to operate over supply voltages from 1.4 to 3.6 V with the maximum quiescent current of 10 µA over the full operating range.
The TMP102 device is ideal for extended temperature measurement in a variety of communication, computer, consumer, environmental, industrial, and instrumentation applications. The device is specified for operation over a temperature range of –40°C to 125°C.
Layout
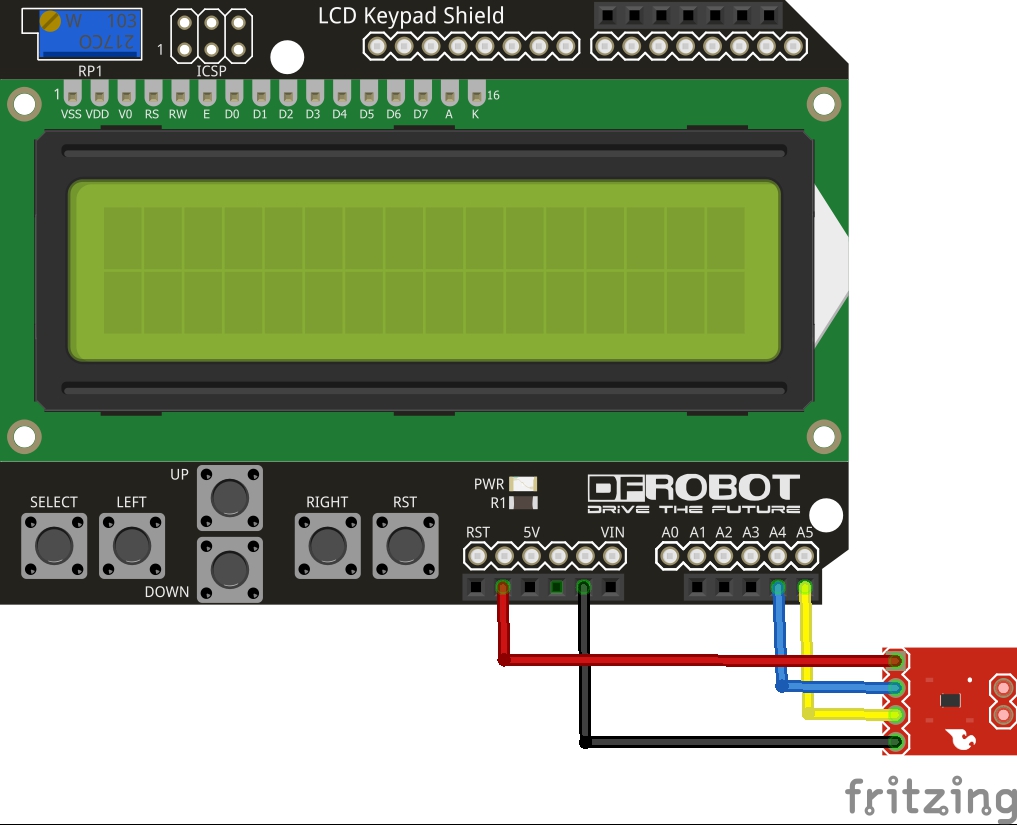
arduino and lcd keypad shield and TMP102 layout
Parts List
Code
This is from the Arduino site with a few tweaks
[codesyntax lang=”cpp”]
#include "Wire.h" #include <LiquidCrystal.h> #define TMP102_I2C_ADDRESS 72 /* This is the I2C address for our chip. This value is correct if you tie the ADD0 pin to ground. See the datasheet for some other values. */ //setup for the LCD keypad shield LiquidCrystal lcd(8, 9, 4, 5, 6, 7); void setup() { Wire.begin(); // start the I2C library Serial.begin(115200); //Start serial communication at 115200 baud lcd.begin(16,2); //line 1 - temperature lcd.setCursor(0,0); lcd.print("TMP102 test"); } void loop() { getTemp102(); delay(1000); //wait 5 seconds before printing our next set of readings. } void getTemp102() { byte firstbyte, secondbyte; //these are the bytes we read from the TMP102 temperature registers int val; float convertedtemp; float correctedtemp; Wire.beginTransmission(TMP102_I2C_ADDRESS); //Say hi to the sensor. Wire.write(0x00); Wire.endTransmission(); Wire.requestFrom(TMP102_I2C_ADDRESS, 2); Wire.endTransmission(); firstbyte = (Wire.read()); secondbyte = (Wire.read()); /*The first byte contains the most significant bits, and the second the less significant */ val = firstbyte; if ((firstbyte & 0x80) > 0) { val |= 0x0F00; } val <<= 4; val |= (secondbyte >> 4); convertedtemp = val*0.0625; correctedtemp = convertedtemp - 5; lcd.setCursor(0,0); //display readings on lcd lcd.setCursor(0,1); lcd.print("Temp: "); lcd.print(correctedtemp); lcd.print(" c"); }
[/codesyntax]
Link
http://www.ti.com/lit/gpn/tmp102